NestJS is a progressive Node.js framework for building efficient, scalable, and maintainable server-side applications. It is built using TypeScript and is inspired by Angular, sharing similar design principles such as modularity, dependency injection, and decorators. This makes NestJS a powerful choice for developers who want to create modern, enterprise-grade backend applications.
Table of Contents
Key Features of NestJS
- Modularity:
- Applications are divided into smaller, reusable modules.
- Modules make the codebase easier to maintain and scale.
- TypeScript First:
- Written entirely in TypeScript, providing strong typing and improved developer productivity.
- Also supports plain JavaScript.
- Dependency Injection:
- Simplifies managing dependencies across your application.
- Makes the code more testable and modular.
- Built-in Support for Middleware and Guards:
- Middleware: Process requests before they reach the controller.
- Guards: Control access to certain routes or resources.
- Integrated with Modern Libraries:
- Works seamlessly with TypeORM, Mongoose, GraphQL, WebSockets, and more.
- Offers first-class support for REST APIs, GraphQL, and microservices.
- Decorator-Based Syntax:
- Simplifies configuration by using decorators like
@Controller
,@Injectable
, and@Module
.
- Simplifies configuration by using decorators like
- Platform-Agnostic:
- Can be used to build applications for REST APIs, GraphQL APIs, WebSocket servers, and microservices.
- Testing Ready:
- Provides tools and utilities for writing unit tests, integration tests, and end-to-end tests.
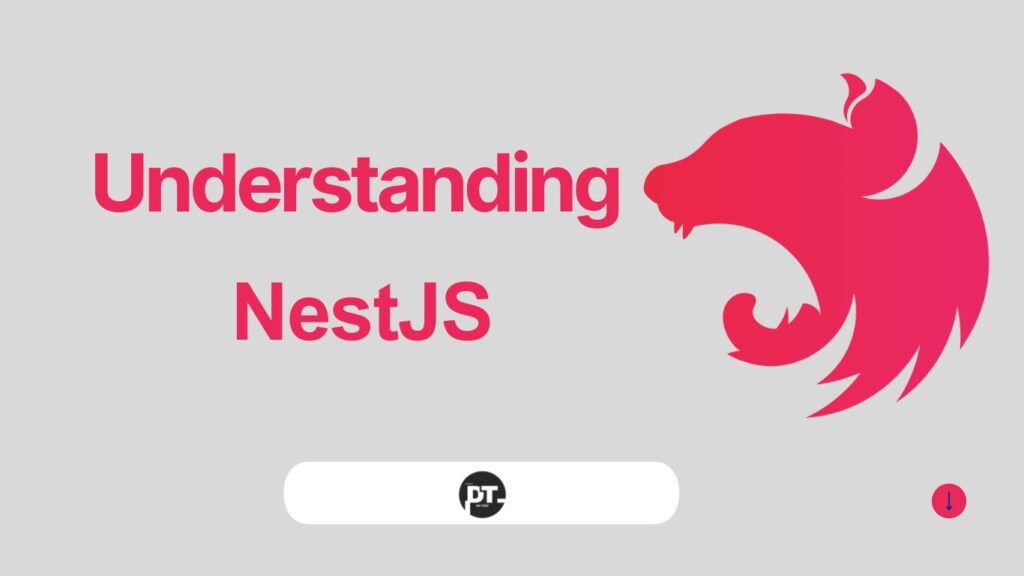
Why Use NestJS?
- Scalability: Modular architecture enables building large-scale applications efficiently.
- Maintainability: Clear separation of concerns and strong typing help in maintaining a clean and robust codebase.
- Enterprise-Grade: Its design and tools make it ideal for enterprise applications.
- Extensibility: Works seamlessly with other libraries and frameworks.
Basic Concepts of NestJS
- Modules:
- The building blocks of a NestJS application.
- Example:
AppModule
serves as the root module.
import { Module } from '@nestjs/common'; import { AppController } from './app.controller'; import { AppService } from './app.service'; @Module({ imports: [], controllers: [AppController], providers: [AppService], }) export class AppModule {}
- Controllers:
- Handle incoming HTTP requests and return responses.
import { Controller, Get } from '@nestjs/common'; @Controller('users') export class UserController { @Get() findAll() { return "List of users"; } }
- Services:
- Contain the business logic of the application and are injected into controllers.
import { Injectable } from '@nestjs/common'; @Injectable() export class UserService { getUsers() { return ['User1', 'User2']; } }
- Providers:
- Used for dependency injection.
- Includes services, repositories, and custom logic.
- Decorators:
- Metadata for configuring classes, methods, and parameters.
- Example:
@Controller
,@Get
,@Post
,@Injectable
.
Advantages of NestJS
- Full TypeScript Support: Boosts productivity and reduces runtime errors.
- Structured Development: Follows clear design patterns for easy collaboration and scaling.
- Extensive Ecosystem: Includes tools for database management, testing, and API building.
- Angular Developers Friendly: Familiar for those experienced with Angular, given its similar design.
Use Cases for NestJS
- RESTful APIs: Build robust REST APIs for modern applications.
- GraphQL APIs: Create powerful and flexible GraphQL-based backend services.
- Microservices: Develop microservices architectures with built-in support for messaging and event-driven systems.
- Real-Time Applications: Handle WebSockets for chat applications, live notifications, or collaborative tools.
- Enterprise Applications: Ideal for large-scale systems that require modularity and maintainability.
Getting Started with NestJS
- Installation:
- Install the CLI:
npm install -g @nestjs/cli
- Create a new project:
nest new project-name
- Install the CLI:
- Run the Application:
npm run start
- Directory Structure:
- Typical structure includes
src
, with modules, controllers, services, and more:src/ ├── app.module.ts ├── app.controller.ts ├── app.service.ts └── main.ts
- Typical structure includes
- First Route: Define a basic route in the
AppController
:@Controller() export class AppController { @Get() getHello(): string { return 'Hello World!'; } }
- Start Adding Features:
- Add modules, controllers, services, and integrate with databases or other APIs as needed.
First Application in Nest JS
Creating your first application in NestJS is straightforward with its CLI (Command Line Interface) and well-structured architecture. This step-by-step guide will walk you through building a simple “Hello World” application in NestJS.
Step 1: Install NestJS CLI
The NestJS CLI simplifies the creation and management of NestJS projects.
Run the following command to install the CLI globally:
npm install -g @nestjs/cli
Verify the installation:
nest --version
Step 2: Create a New Project
Create a new NestJS application using the CLI:
nest new first-app
- You will be prompted to choose a package manager (e.g., npm or yarn). Choose your preference.
- Navigate into the project directory:
cd first-app
Step 3: Understand the Project Structure
The CLI generates a structured application:
first-app/
├── src/
│ ├── app.controller.ts # Handles incoming requests and responses
│ ├── app.module.ts # Root module of the application
│ ├── app.service.ts # Contains business logic
│ ├── main.ts # Entry point of the application
├── test/ # Contains unit test files
├── package.json # Manages dependencies
├── tsconfig.json # TypeScript configuration
Step 4: Start the Application
Run the development server:
npm run start
The application will run on http://localhost:3000. Open it in your browser to see the default “Hello World!” response.
Step 5: Modify the Default Application
Update the App Controller
The app.controller.ts
file contains the logic for handling incoming requests. By default, it has a getHello()
method.
Modify it to return a custom message:
import { Controller, Get } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
getHello(): string {
return 'Welcome to your first NestJS application!';
}
}
Update the App Service
The app.service.ts
file contains the business logic for the AppController
.
You can modify it or leave it unchanged for now:
import { Injectable } from '@nestjs/common';
@Injectable()
export class AppService {
getHello(): string {
return 'Welcome to your first NestJS application!';
}
}
Step 6: Add a New Route
Let’s add a new route to handle a different URL.
Modify app.controller.ts
:
Add a new @Get()
method for a /greet
route:
import { Controller, Get } from '@nestjs/common';
@Controller()
export class AppController {
@Get()
getHello(): string {
return 'Welcome to your first NestJS application!';
}
@Get('greet')
greet(): string {
return 'Hello from the /greet route!';
}
}
- Visit http://localhost:3000/greet to see the new response.
Step 7: Add a Service Dependency
NestJS uses dependency injection to keep the code modular and testable.
Update the Service (app.service.ts
):
Add a method to return a greeting:
@Injectable()
export class AppService {
getHello(): string {
return 'Welcome to your first NestJS application!';
}
getGreeting(): string {
return 'This is a greeting from the service!';
}
}
Use the Service in the Controller:
Inject the service into the controller and use the new method:
import { Controller, Get } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
getHello(): string {
return this.appService.getHello();
}
@Get('greet')
greet(): string {
return this.appService.getGreeting();
}
}
Step 8: Run the Application
Restart the server if it’s not running:
npm run start
Test the endpoints:
- http://localhost:3000 → Returns: “Welcome to your first NestJS application!”
- http://localhost:3000/greet → Returns: “This is a greeting from the service!”
Step 9: Add Middleware (Optional)
Middleware allows you to process requests before they reach a controller.
Create Middleware:
nest generate middleware logger
NestJS will create a new file logger.middleware.ts
. Update it:
import { Injectable, NestMiddleware } from '@nestjs/common';
import { Request, Response, NextFunction } from 'express';
@Injectable()
export class LoggerMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: NextFunction) {
console.log(`[${req.method}] ${req.url}`);
next();
}
}
Apply Middleware:
Modify app.module.ts
to apply the middleware:
import { Module, MiddlewareConsumer } from '@nestjs/common';
import { AppController } from './app.controller';
import { AppService } from './app.service';
import { LoggerMiddleware } from './logger.middleware';
@Module({
imports: [],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {
configure(consumer: MiddlewareConsumer) {
consumer.apply(LoggerMiddleware).forRoutes('*');
}
}
Now, every request will log the method and URL to the console.
You’ve built and customized your first NestJS application! Here’s what you’ve learned:
- Creating a new NestJS project.
- Understanding the basic structure.
- Adding and modifying routes.
- Using services for business logic.
- Adding middleware for request processing.
NestJS’s modular and extensible design makes it easy to build complex, enterprise-grade applications. You can now explore features like database integration, GraphQL, or building microservices!
Key Differences Between NestJS and Node.js
Feature | Node.js | NestJS |
---|---|---|
Type | Runtime Environment | Framework (built on top of Node.js) |
Core Technology | JavaScript (or TypeScript) runtime for building I/O-driven applications | Built with TypeScript (but also supports JavaScript), built on top of Node.js |
Use Cases | Web servers, RESTful APIs, real-time apps, microservices | Backend web applications, APIs, GraphQL, microservices, enterprise applications |
Design Pattern | Event-driven, callback-based, single-threaded with event-loop | Modular, uses decorators, follows MVC-like architecture |
Architecture | Minimal and flexible, developers define their structure | Opinionated structure with built-in support for controllers, services, and modules |
Dependency Injection | Manual (you handle dependencies yourself) | Built-in dependency injection system for services and controllers |
Learning Curve | Steeper for beginners (since you define much of the structure yourself) | Easier for developers familiar with Angular or TypeScript |
Testing | Manual setup for testing (using Mocha, Jest, etc.) | Built-in testing support, integrates easily with Jest and other libraries |
Community & Ecosystem | Large community with numerous available packages (via npm) | Growing community, fewer packages compared to Node.js, but excellent for large-scale applications |
Scalability | Good, but often requires custom solutions for complex architectures | Excellent scalability with support for microservices, GraphQL, and robust architecture patterns |
Performance | High performance for I/O-bound tasks (due to non-blocking nature) | Slight overhead due to abstraction, but still performs well for most applications |
When to Use Node.js vs NestJS?
Use Node.js when:
- You need low-level control over the application and want to build something from scratch without any structural constraints.
- You want to build applications that focus heavily on real-time data, such as chat applications or live updates (e.g., WebSockets).
- You prefer working directly with JavaScript or have performance-critical applications that require fine-tuned optimization.
- You need a lightweight solution where you prefer minimal abstraction and direct control over the request-response cycle.
Use NestJS when:
- You are building large-scale applications that require clean architecture, maintainability, and scalability.
- You prefer using TypeScript or come from an Angular background (because of the similar design patterns and concepts).
- You want to leverage built-in features like dependency injection, modular structure, and decorators for routing and services.
- You need to build applications that integrate easily with GraphQL, microservices, or require enterprise-grade architecture.
5. Example: A Simple API
Here’s a basic comparison of how a simple API might look in both Node.js and NestJS:
Node.js (Express Example)
javascriptCopy codeconst express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello from Node.js!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
NestJS (Using Controllers and Services)
- app.controller.ts (Controller):
typescriptCopy codeimport { Controller, Get } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
getHello(): string {
return this.appService.getHello();
}
}
- app.service.ts (Service):
typescriptCopy codeimport { Injectable } from '@nestjs/common';
@Injectable()
export class AppService {
getHello(): string {
return 'Hello from NestJS!';
}
}
- main.ts (Entry Point):
typescriptCopy codeimport { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
async function bootstrap() {
const app = await NestFactory.create(AppModule);
await app.listen(3000);
}
bootstrap();
Conclusion
NestJS combines the power of TypeScript, modular architecture, and a robust ecosystem to create scalable, maintainable, and high-performance server-side applications. Whether you’re building APIs, microservices, or real-time systems, NestJS is an excellent choice for developers looking for a modern, full-featured framework.
[…] Understanding NestJS: A Powerful Node.js Framework […]
[…] PostgreSQL with Nest JS offers several benefits, as the database’s strengths complement the NestJS framework’s […]
[…] in NestJS are defined in controllers using decorators like @Get, @Post, @Put, @Delete, […]
[…] following these guidelines, you can effectively manage sessions in a NestJS application, ensuring scalability, security, and seamless user […]